Using Tkinter, to create a file explorer in Python.
Using Python’s Tkinter, OS, and shutil modules, we’ll create a GUI-based File Explorer in this Python project.
It’s a beginner-level project, so you’ll need a basic understanding of all the libraries, and you’ll get to use them in real life with this project. Let’s get this coding started!
About File Managers:
A file manager is a piece of software that allows a user to handle various types of files on a computer.
The File Explorer, which is included in all operating systems, is the most widely recognized sort of file manager.
About the python file explorer project:
The goal is to design a File Manager with a graphical user interface.
You’ll need a basic understanding of Tkinter, OS, and shutil libraries to develop this.
Structure of the Project’s Files
To make this python file explorer project, you’ll need to follow these steps:
- Importing the required modules is the first step.
- Â Defining the functions of all the buttons as well as the GUI window’s variables.
- Setting up the window, inserting the components, and closing it.
Project Prerequisites:
The following libraries will be required to complete this python file explorer project:
1. Tkinter is used to develop the graphical user interface (GUI).
2. Operating System (OS) – To operate on a file and its path.
3. Shutil is a command that allows you to copy or move a file.
1. Importing all required modules:
from tkinter import *
import tkinter.filedialog as fd
import tkinter.messagebox as mb
import os
import shutil
Explanation:
- The from tkinter import * statement imports everything from the tkinter library’s init file.
- The filedialog module contains routines for opening and saving dialogues.
- The messagebox module is a set of functions that show various types of messages using various icons.
2. Defining the functions of all the buttons and the variables for the GUI window:
# Creating the backend functions for python file explorer project
def open_file():
file = fd.askopenfilename(title='Choose a file of any type', filetypes=[("All files", "*.*")])
os.startfile(os.path.abspath(file))
def copy_file():
file_to_copy = fd.askopenfilename(title='Choose a file to copy', filetypes=[("All files", "*.*")])
dir_to_copy_to = fd.askdirectory(title="In which folder to copy to?")
try:
shutil.copy(file_to_copy, dir_to_copy_to)
mb.showinfo(title='File copied!', message='Your desired file has been copied to your desired location')
except:
mb.showerror(title='Error!', message='We were unable to copy your file to the desired location. Please try again')
def delete_file():
file = fd.askopenfilename(title='Choose a file to delete', filetypes=[("All files", "*.*")])
os.remove(os.path.abspath(file))
mb.showinfo(title='File deleted', message='Your desired file has been deleted')
def rename_file():
file = fd.askopenfilename(title='Choose a file to rename', filetypes=[("All files", "*.*")])
rename_wn = Toplevel(root)
rename_wn.title("Rename the file to")
rename_wn.geometry("250x70"); rename_wn.resizable(0, 0)
Label(rename_wn, text='What should be the new name of the file?', font=("Times New Roman", 10)).place(x=0, y=0)
new_name = Entry(rename_wn, width=40, font=("Times New Roman", 10))
new_name.place(x=0, y=30)
new_file_name = os.path.join(os.path.dirname(file), new_name.get()+os.path.splitext(file)[1])
os.rename(file, new_file_name)
mb.showinfo(title="File Renamed", message='Your desired file has been renamed')
def open_folder():
folder = fd.askdirectory(title="Select Folder to open")
os.startfile(folder)
def delete_folder():
folder_to_delete = fd.askdirectory(title='Choose a folder to delete')
os.rmdir(folder_to_delete)
mb.showinfo("Folder Deleted", "Your desired folder has been deleted")
def move_folder():
folder_to_move = fd.askdirectory(title='Select the folder you want to move')
mb.showinfo(message='You just selected the folder to move, now please select the desired destination where you want to move the folder to')
destination = fd.askdirectory(title='Where to move the folder to')
try:
shutil.move(folder_to_move, destination)
mb.showinfo("Folder moved", 'Your desired folder has been moved to the location you wanted')
except:
mb.showerror('Error', 'We could not move your folder. Please make sure that the destination exists')
def list_files_in_folder():
i = 0
folder = fd.askdirectory(title='Select the folder whose files you want to list')
files = os.listdir(os.path.abspath(folder))
list_files_wn = Toplevel(root)
list_files_wn.title(f'Files in {folder}')
list_files_wn.geometry('250x250')
list_files_wn.resizable(0, 0)
listbox = Listbox(list_files_wn, selectbackground='SteelBlue', font=("Georgia", 10))
listbox.place(relx=0, rely=0, relheight=1, relwidth=1)
scrollbar = Scrollbar(listbox, orient=VERTICAL, command=listbox.yview)
scrollbar.pack(side=RIGHT, fill=Y)
listbox.config(yscrollcommand=scrollbar.set)
while i < len(files):
listbox.insert(END, files[i])
i += 1
# Defining the variables
title = 'IAMNK File Manager'
background = '#C80D0D'
button_font = ("Times New Roman", 13)
button_background = '#ffde59'
Explanation:
- The components of the filedialog module utilised in this project are as follows:
- To pick a file, use the askfilename function, with title title and filetypes filetypes.
- To choose a folder, use the askdirectory function.
- Both of these functions keep track of the file’s path.
- The components of the OS library utilised in this project are as follows:
- The path module has the following functions:
- The abspath function returns the path of the file sent in as an argument.
- The join function joins two portions of a file, usually the name and extension.
- The dirname function returns the name of the directory in which a file is stored.
- The splittext function is used to split a file’s name into a list with the file’s name and extensions as separate items.
- The path module has the following functions:
- The init module has the following functions:
- To rename a file or folder, use the rename function.
- To open a file in an external application, use the startfile function.
- To delete a whole directory, use the rmdir function.
- To delete a file, use the remove function.
- The following are the components of the Shutil library that were utilised in this project:
- The copy function copies a file from its source location, src, to the computer’s desired destination, dst.
- The move function is used to move a file from one location to another, from source to destination.
Note: In the next step, all of the tkinter aspects in this step will be described.
3. Creating the window, putting the components in place, and finishing it:
# Initializing the window
root = Tk()
root.title(title)
root.geometry('250x400')
root.resizable(0, 0)
root.config(bg=background)
# Creating and placing the components in the window
Label(root, text=title, font=("Comic Sans MS", 15), bg=background, wraplength=250).place(x=20, y=0)
Button(root, text='Open a file', width=20, font=button_font, bg=button_background, command=open_file).place(x=30, y=50)
Button(root, text='Copy a file', width=20, font=button_font, bg=button_background, command=copy_file).place(x=30, y=90)
Button(root, text='Rename a file', width=20, font=button_font, bg=button_background, command=rename_file).place(x=30, y=130)
Button(root, text='Delete a file', width=20, font=button_font, bg=button_background, command=delete_file).place(x=30, y=170)
Button(root, text='Open a folder', width=20, font=button_font, bg=button_background, command=open_folder).place(x=30, y=210)
Button(root, text='Delete a folder', width=20, font=button_font, bg=button_background, command=delete_folder).place(x=30, y=250)
Button(root, text='Move a folder', width=20, font=button_font, bg=button_background, command=move_folder).place(x=30, y=290)
Button(root, text='List all files in a folder', width=20, font=button_font, bg=button_background,
command=list_files_in_folder).place(x=30, y=330)
# Finalizing the window
root.update()
root.mainloop()
Explanation:
- The GUI window is assigned to a variable using the Tk() class.
- The.title() method is used to give the window a title.
- The.geometry() method is used to define the window’s initial geometry.
- The.resizable() method is used to give the user the option of resizing or not resizing a window.
- The.config() method is used to configure the object’s extra characteristics.
- The Label class creates a Label widget in the window, which is a frame for displaying static text. It has the following methods and attributes:
- The widget’s parent window is specified by the master attribute.
- The text attribute is used to specify the text that will be shown in the widget.
- The font element is used to provide the text’s font name, font size, and bold/italic.
- The bg attribute specifies the widget’s background colour.
- The wraplength property specifies how many pixels the text will wrap to next line after.
- The Button class adds a button to its parent element that, when pressed, performs a function.
It has the following characteristics:- The widget’s width attribute specifies the widget’s length in pixels.
- When the button is pressed, the command attribute is used to specify the function that will be performed.
- The other characteristics have already been mentioned.
- The Toplevel class is similar to the Frame widget, except that its child widgets are always displayed in a new window.
- This class has the same methods and characteristics as the Tk() class.
- In the GUI window, the Entry class is used to add an input field.
It employs the following strategies:- The textvariable property is used to create a StringVar(string), IntVar(integer), or DoubleVar(float) variable to store or update the value in the Entry widget, as well as to give it a type [as shown in brackets against the __Var words].
- The class’s other characteristics have already been explored.
- The.get() method is used to retrieve the user’s input.
This method does not accept any parameters.
- The Listbox class is used to add a multiline, static textbox to the window from which the user can choose items.
- The selectbackground attribute is used to define the colour of the selected background.
- The yscroll command attribute is used to specify the scrollbar that will be used to manage the widget’s up-and-down scrolling.
- The other characteristics have already been mentioned.
- To insert an element or elements, *elements, at the index index, use the.insert(index, *elements) method.
- An appropriate tkinter constant or an integer can be used as the index argument.
- The.yview() method is used to create a scrollbar that allows you to see the up and down of the widget you’re working with.
- The Scrollbar class is used to add a scrollbar to a widget’s parent that regulates the parent’s movement.
- The orient parameter specifies the orientation of the scrollbar on the window.
- The other characteristics have already been mentioned.
- To connect the scrollbar to another widget, use the.set() method.
The Complete Code:
from tkinter import *
import tkinter.filedialog as fd
import tkinter.messagebox as mb
import os
import shutil
# Creating the backend functions
def open_file():
file = fd.askopenfilename(title='Choose a file of any type', filetypes=[("All files", "*.*")])
os.startfile(os.path.abspath(file))
def copy_file():
file_to_copy = fd.askopenfilename(title='Choose a file to copy', filetypes=[("All files", "*.*")])
dir_to_copy_to = fd.askdirectory(title="In which folder to copy to?")
try:
shutil.copy(file_to_copy, dir_to_copy_to)
mb.showinfo(title='File copied!', message='Your desired file has been copied to your desired location')
except:
mb.showerror(title='Error!', message='We were unable to copy your file to the desired location. Please try again')
def delete_file():
file = fd.askopenfilename(title='Choose a file to delete', filetypes=[("All files", "*.*")])
os.remove(os.path.abspath(file))
mb.showinfo(title='File deleted', message='Your desired file has been deleted')
def rename_file():
file = fd.askopenfilename(title='Choose a file to rename', filetypes=[("All files", "*.*")])
rename_wn = Toplevel(root)
rename_wn.title("Rename the file to")
rename_wn.geometry("250x70"); rename_wn.resizable(0, 0)
Label(rename_wn, text='What should be the new name of the file?', font=("Times New Roman", 10)).place(x=0, y=0)
new_name = Entry(rename_wn, width=40, font=("Times New Roman", 10))
new_name.place(x=0, y=30)
new_file_name = os.path.join(os.path.dirname(file), new_name.get()+os.path.splitext(file)[1])
os.rename(file, new_file_name)
mb.showinfo(title="File Renamed", message='Your desired file has been renamed')
def open_folder():
folder = fd.askdirectory(title="Select Folder to open")
os.startfile(folder)
def delete_folder():
folder_to_delete = fd.askdirectory(title='Choose a folder to delete')
os.rmdir(folder_to_delete)
mb.showinfo("Folder Deleted", "Your desired folder has been deleted")
def move_folder():
folder_to_move = fd.askdirectory(title='Select the folder you want to move')
mb.showinfo(message='You just selected the folder to move, now please select the desired destination where you want to move the folder to')
destination = fd.askdirectory(title='Where to move the folder to')
try:
shutil.move(folder_to_move, destination)
mb.showinfo("Folder moved", 'Your desired folder has been moved to the location you wanted')
except:
mb.showerror('Error', 'We could not move your folder. Please make sure that the destination exists')
def list_files_in_folder():
folder = fd.askdirectory(title='Select the folder whose files you want to list')
files = os.listdir(str(os.path.abspath(folder)))
list_files_wn = Toplevel(root)
list_files_wn.title('Files in your selected folder')
list_files_wn.geometry('150x250')
list_files_wn.resizable(0, 0)
listbox = Listbox(list_files_wn, selectbackground='SteelBlue', font=("Georgia", 10), height=15, width=20)
scrollbar = Scrollbar(listbox, orient=VERTICAL, command=listbox.yview)
scrollbar.pack(side=RIGHT)
listbox.config(yscrollcommand=scrollbar.set)
listbox.place(x=10, y=10)
for file in list(files):
listbox.insert(END, file)
# Defining the variables
title = 'IAMNK File Manager'
background = '#C80D0D'
button_font = ("Times New Roman", 13)
button_background = '#ffde59'
# Initializing the window
root = Tk()
root.title(title)
root.geometry('250x400')
root.resizable(0, 0)
root.config(bg=background)
# Creating and placing the components in the window
Label(root, text=title, font=("Comic Sans MS", 15), bg=background, wraplength=250).place(x=20, y=0)
Button(root, text='Open a file', width=20, font=button_font, bg=button_background, command=open_file).place(x=30, y=50)
Button(root, text='Copy a file', width=20, font=button_font, bg=button_background, command=copy_file).place(x=30, y=90)
Button(root, text='Rename a file', width=20, font=button_font, bg=button_background, command=rename_file).place(x=30, y=130)
Button(root, text='Delete a file', width=20, font=button_font, bg=button_background, command=delete_file).place(x=30, y=170)
Button(root, text='Open a folder', width=20, font=button_font, bg=button_background, command=open_folder).place(x=30, y=210)
Button(root, text='Delete a folder', width=20, font=button_font, bg=button_background, command=delete_folder).place(x=30, y=250)
Button(root, text='Move a folder', width=20, font=button_font, bg=button_background, command=move_folder).place(x=30, y=290)
Button(root, text='List all files in a folder', width=20, font=button_font, bg=button_background,
command=list_files_in_folder).place(x=30, y=330)
# Finalizing the window
root.update()
root.mainloop()
The Final Output:
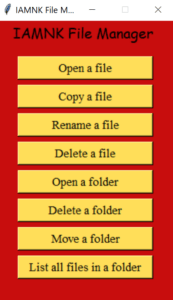
Congratulations,
I think the Python File Manager is really cool, and I hope you enjoyed the process as much as I did.
As a result, if you like what you’ve read, please leave a comment and follow me on YouTube, Facebook, Twitter, and LinkedIn. Don’t forget to subscribe to my channel and clicking the bell icon.